概要
Pythonで画像を簡単にリサイズできるGUIプログラムを作成する方法を紹介します。このプログラムは、ドラッグアンドドロップで画像ファイルを入力し、指定されたサイズと形式にリサイズして保存できます。対応する画像形式は、PNG、JPG、BMP、GIF、TIFFです。リサイズする際にはアスペクト比を保持し、複数ページのTIFF画像にも対応しています。
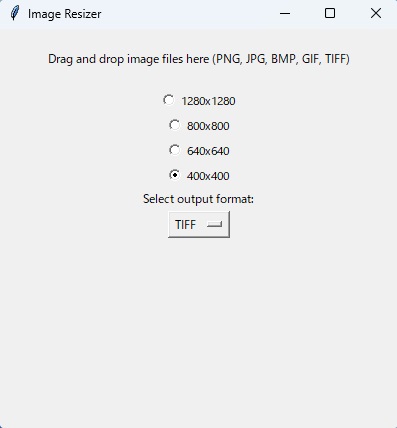
使用例
このプログラムを使用することで、以下のような用途に役立ちます:
- ウェブサイトにアップロードする画像のサイズを統一したい場合
- 高解像度の画像を手軽に縮小したい場合
- さまざまな画像形式を統一したい場合
例えば、ブログやオンラインショップで使用する画像を統一サイズにリサイズして、ページの読み込み速度を向上させることができます。
必要なPythonライブラリとインストール方法
このプログラムを実行するためには、以下のPythonライブラリが必要です:
- tkinterdnd2
- Pillow (PIL fork)
これらのライブラリは、以下のコマンドを使用してインストールできます:
pip install tkinterdnd2 pillow
使用手順
- 必要なライブラリをインストールします。
- 下記のコードをコピーして、テキストエディタ(例:メモ帳)に貼り付け、「image_resizer.py」という名前で保存します。
- ターミナルやコマンドプロンプトを開き、保存したファイルのディレクトリに移動します。
- 以下のコマンドを実行してプログラムを起動します:
python image_resizer.py
- プログラムが起動したら、リサイズしたい画像ファイルをドラッグアンドドロップします。
- リサイズ後の画像が指定した形式で保存されます。
注意点
- リサイズする画像のアスペクト比は保持されます。指定したサイズに収まるように縮小されますが、元のアスペクト比は維持されます。
- 複数ページのTIFF画像の場合、各ページが個別のファイルとして保存されます。
- 入力されたファイルが対応していない形式の場合、エラーメッセージが表示されます。
プログラム
下記のコードをメモ帳などに丸々コピーして、pythonファイル(image_resizer.py)にしてください。
import tkinter as tk
from tkinterdnd2 import TkinterDnD, DND_FILES
from PIL import Image, UnidentifiedImageError
import os
# Define sizes for resizing
SIZES = {
"1280x1280": (1280, 1280),
"800x800": (800, 800),
"640x640": (640, 640),
"400x400": (400, 400),
}
# Supported image formats
SUPPORTED_FORMATS = ('.png', '.jpg', '.jpeg', '.bmp', '.gif', '.tiff')
OUTPUT_FORMATS = ["PNG", "JPG", "BMP", "GIF", "TIFF"]
# Create a function to generate a unique filename
def generate_unique_filename(base, ext):
counter = 1
while True:
new_filename = f"{base}_resized_{counter:03d}{ext}"
if not os.path.exists(new_filename):
return new_filename
counter += 1
# Create a function to resize and save the image
def process_image(file_path, size, output_format):
try:
with Image.open(file_path) as img:
if img.format == 'TIFF' and getattr(img, "n_frames", 1) > 1:
for i in range(img.n_frames):
img.seek(i)
frame = img.copy()
frame.thumbnail(size)
base, _ = os.path.splitext(file_path)
output_ext = "." + output_format.lower()
output_path = generate_unique_filename(f"{base}_{i+1}", output_ext)
frame.save(output_path, output_format)
print(f"Saved resized image to: {output_path}")
else:
img.thumbnail(size)
base, _ = os.path.splitext(file_path)
output_ext = "." + output_format.lower()
output_path = generate_unique_filename(base, output_ext)
img.save(output_path, output_format)
print(f"Saved resized image to: {output_path}")
except UnidentifiedImageError:
print(f"Unsupported image format: {file_path}")
error_label.config(text=f"Unsupported image format: {file_path}")
except Exception as e:
print(f"Error processing {file_path}: {e}")
error_label.config(text=f"Error processing {file_path}: {e}")
# Create a function to handle dropped files
def drop(event):
error_label.config(text="")
files = root.tk.splitlist(event.data)
size = SIZES[selected_size.get()]
output_format = selected_format.get()
for file_path in files:
if file_path.lower().endswith(SUPPORTED_FORMATS):
process_image(file_path, size, output_format)
else:
error_label.config(text=f"Unsupported file format: {file_path}")
print(f"Unsupported file format: {file_path}")
# Create the main application window
root = TkinterDnD.Tk()
root.title("Image Resizer")
root.geometry("400x400")
# Variable to store the selected size
selected_size = tk.StringVar(root, value="1280x1280")
# Variable to store the selected output format
selected_format = tk.StringVar(root, value="JPG")
# Add a label to guide the user
label = tk.Label(root, text="Drag and drop image files here (PNG, JPG, BMP, GIF, TIFF)", pady=20)
label.pack()
# Add radio buttons for size selection
for text, value in SIZES.items():
tk.Radiobutton(root, text=text, variable=selected_size, value=text).pack()
# Add a dropdown menu for output format selection
format_label = tk.Label(root, text="Select output format:")
format_label.pack()
format_menu = tk.OptionMenu(root, selected_format, *OUTPUT_FORMATS)
format_menu.pack()
# Add a label to display error messages
error_label = tk.Label(root, text="", fg="red", pady=10)
error_label.pack()
# Bind the drop event
root.drop_target_register(DND_FILES)
root.dnd_bind('<<Drop>>', drop)
# Run the application
root.mainloop()
あるいは、下のテキストファイルをダウンロードし、「.txt」を「.py」に変えることでそのまま使えます。
まとめ
このPythonプログラムを使用することで、さまざまな画像形式を簡単にリサイズし、指定した形式で保存することができます。ウェブサイトやブログ、オンラインショップで画像を統一する際に非常に便利です。ぜひこのプログラムを活用して、画像処理の効率を向上させてください。